Table Of Content
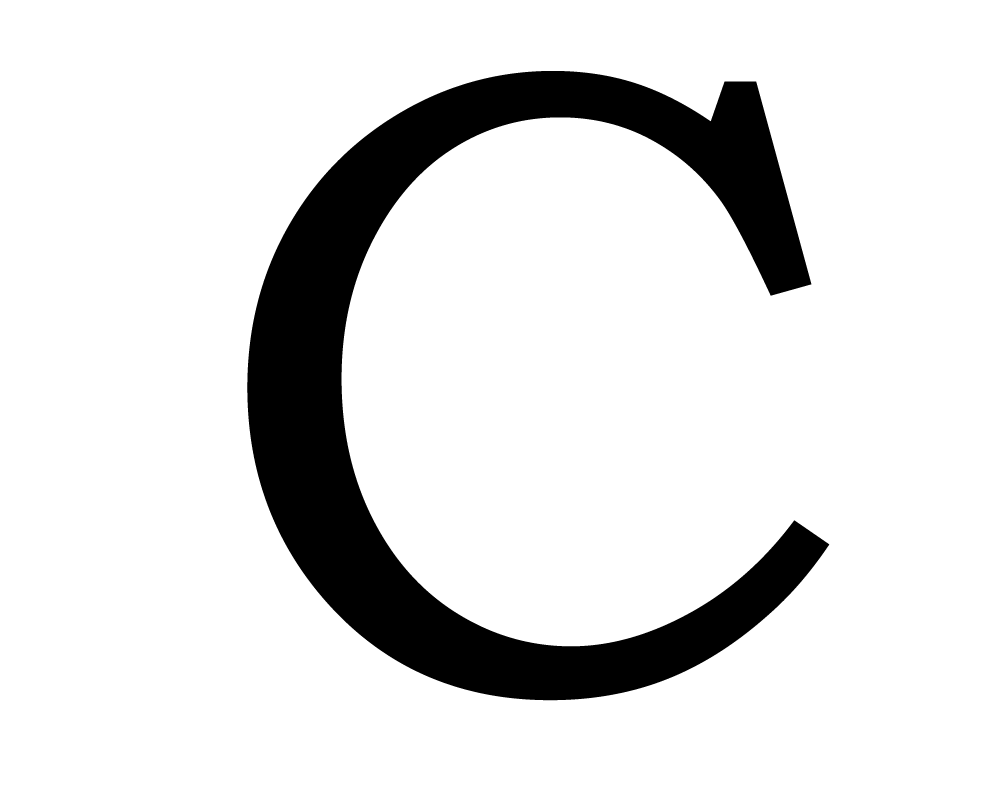
For example, the backslashes in "This string contains \"double quotes\"." indicate (to the compiler) that the inner pair of quotes are intended as an actual part of the string, rather than the default reading as a delimiter (endpoint) of the string itself. In C, string literals are surrounded by double quotes (") (e.g., "Hello world!") and are compiled to an array of the specified char values with an additional null terminating character (0-valued) code to mark the end of the string. This ensures that further attempts to dereference the pointer, on most systems, will crash the program. If this is not done, the variable becomes a dangling pointer which can lead to a use-after-free bug. However, if the pointer is a local variable, setting it to NULL does not prevent the program from using other copies of the pointer. Local use-after-free bugs are usually easy for static analyzers to recognize.
Parasoft unveils safety testing tool for C and C++ apps
Encodings lacking these features are likely to prove incompatible with the standard library functions; encoding-aware string functions are often used in such cases. The type system in C is static and weakly typed, which makes it similar to the type system of ALGOL descendants such as Pascal.[36] There are built-in types for integers of various sizes, both signed and unsigned, floating-point numbers, and enumerated types (enum). There are also derived types including arrays, pointers, records (struct), and unions (union). One of the aims of the C standardization process was to produce a superset of K&R C, incorporating many of the subsequently introduced unofficial features. The standards committee also included several additional features such as function prototypes (borrowed from C++), void pointers, support for international character sets and locales, and preprocessor enhancements.
Jump statements
Pass-by-reference is simulated in C by explicitly passing pointers to the thing being referenced. A successor to the programming language B, C was originally developed at Bell Labs by Ritchie between 1972 and 1973 to construct utilities running on Unix. It was applied to re-implementing the kernel of the Unix operating system.[8] During the 1980s, C gradually gained popularity. It has become one of the most widely used programming languages,[9][10] with C compilers available for practically all modern computer architectures and operating systems. An implementation of C providing all of the standard library functions is called a hosted implementation.
Pointers
In some cases, we are only interested in the memory address itself, and we might just want to pass that address around, for example to a function that doesn’t care how the data itself is arranged. If we use the void pointer, we can simply assign to and from it without any explicit type cast necessary, which can help us keep the code a bit cleaner. Strings, both constant and variable, can be manipulated without using the standard library. However, the library contains many useful functions for working with null-terminated strings. Note that storage specifiers apply only to functions and objects; other things such as type and enum declarations are private to the compilation unit in which they appear. In addition to the standard integer types, there may be other "extended" integer types, which can be used for typedefs in standard headers.
Global structure
The use of other backslash escapes is not defined by the C standard, although compiler vendors often provide additional escape codes as language extensions. One of these is the escape sequence \e for the escape character with ASCII hex value 1B which was not added to the C standard due to lacking representation in other character sets (such as EBCDIC). Variables declared within a block by default have automatic storage, as do those explicitly declared with the auto[note 2] or register storage class specifiers. The auto and register specifiers may only be used within functions and function argument declarations; as such, the auto specifier is always redundant. Objects declared outside of all blocks and those explicitly declared with the static storage class specifier have static storage duration.
Why the C programming language still rules
In conditional contexts, null pointer values evaluate to false, while all other pointer values evaluate to true. The C standard library is small compared to the standard libraries of some other languages. The C library provides a basic set of mathematical functions, string manipulation, type conversions, and file and console-based I/O. It does not include a standard set of "container types" like the C++ Standard Template Library, let alone the complete graphical user interface (GUI) toolkits, networking tools, and profusion of other functionality that Java and the .NET Framework provide as standard. The main advantage of the small standard library is that providing a working ISO C environment is much easier than it is with other languages, and consequently porting C to a new platform is comparatively easy. Structures and unions in C are defined as data containers consisting of a sequence of named members of various types.
Lowering U.S. and NC Flags to Half-Staff in Honor of Former NC Judge John C. Martin - NC.gov
Lowering U.S. and NC Flags to Half-Staff in Honor of Former NC Judge John C. Martin.
Posted: Thu, 25 Apr 2024 22:10:41 GMT [source]
Reading the subscripts from left to right, array2d is an array of length ROWS, each element of which is an array of COLUMNS integers. The primary facility for accessing the values of the elements of an array is the array subscript operator. To access the i-indexed element of array, the syntax would be array[i], which refers to the value stored in that array element. The book introduced the "Hello, World!" program, which prints only the text "hello, world", as an illustration of a minimal working C program. Since then, many texts have followed that convention for introducing a programming language. In cases where code must be compilable by either standard-conforming or K&R C-based compilers, the __STDC__ macro can be used to split the code into Standard and K&R sections to prevent the use on a K&R C-based compiler of features available only in Standard C.
Typically, the failure symptoms appear in a portion of the program unrelated to the code that causes the error, making it difficult to diagnose the failure. Such issues are ameliorated in languages with automatic garbage collection. The basic C execution character set contains the same characters, along with representations for alert, backspace, and carriage return. Run-time support for extended character sets has increased with each revision of the C standard. In early versions of C, only functions that return types other than int must be declared if used before the function definition; functions used without prior declaration were presumed to return type int. However, the built-in functions must behave like ordinary functions in accordance with ISO C. The main implication is that the program must be able to create a pointer to these functions by taking their address, and invoke the function by means of that pointer.

Control structures
For more precise specification of width, programmers can and should use typedefs from the standard header stdint.h. We have improved the exposition of critical features, such as pointers, that are central to C programming. We have refined the original examples, and have added new examples in several chapters. For instance, the treatment of complicated declarations is augmented by programs that convert declarations into words and vice versa. As before, all examples have been tested directly from the text, which is in machine-readable form. There are also compilers, libraries, and operating system level mechanisms for performing actions that are not a standard part of C, such as bounds checking for arrays, detection of buffer overflow, serialization, dynamic memory tracking, and automatic garbage collection.
(A workaround for this was to allocate the array with an additional "row vector" of pointers to the columns.) C99 introduced "variable-length arrays" which address this issue. Separate tools such as Unix's lint utility were developed that (among other things) could check for consistency of function use across multiple source files. Thompson wanted a programming language for developing utilities for the new platform. Instead, he created a cut-down version of the recently developed systems programming language called BCPL. In C, all executable code is contained within subroutines (also called "functions", though not in the sense of functional programming). Function parameters are passed by value, although arrays are passed as pointers, i.e. the address of the first item in the array.
An incomplete type is a structure or union type whose members have not yet been specified, an array type whose dimension has not yet been specified, or the void type (the void type cannot be completed). Such a type may not be instantiated (its size is not known), nor may its members be accessed (they, too, are unknown); however, the derived pointer type may be used (but not dereferenced). The type qualifier const indicates that a value does not change once it has been initialized. Attempting to modify a const qualified value yields undefined behavior, so some C compilers store them in rodata or (for embedded systems) in read-only memory (ROM).
The declaration-list declares variables to be used in that scope, and the statement-list are the actions to be performed. Brackets define their own scope, and variables defined inside those brackets will be automaticallydeallocated at the closing bracket. Declarations and statements can be freely intermixed within a compound statement (as in C++).
Correction and Clarification of C.26 Rapid Mission Design Studies for Mars Sample Return - Science@NASA
Correction and Clarification of C.26 Rapid Mission Design Studies for Mars Sample Return.
Posted: Fri, 26 Apr 2024 17:32:24 GMT [source]
C is an imperative procedural language, supporting structured programming, lexical variable scope, and recursion, with a static type system. It was designed to be compiled to provide low-level access to memory and language constructs that map efficiently to machine instructions, all with minimal runtime support. Despite its low-level capabilities, the language was designed to encourage cross-platform programming.
No comments:
Post a Comment